Hill 88
Hill 88 is a favorite walk when the Marin headlands are free from fog. Starting from Fort Cronkhite the Coastal Trail winds up to Hill 88 at 960ft, past various WWII era coastal defenses. Cross the ridge and descend down Wolf Ridge Trail and Miwok Trail to complete a 4.5 mile loop.
Rodeo Beach viewed from the Coastal Trail. As you ascend there are views of the Golden Gate Bridge and San Francisco.
Battery Townsley, one of several coastal defenses passed on the walk.
View of Mount Tamalpais as you begin to descend Wolf Ridge Trail.
(4.56 miles, total elevation gain 1,142 feet, 1 hour 38 minutes (average 2.79 mph), view in Google Earth)
Hike starts at: 37.832683, -122.526217.
Updated 2021-09-07 17:55:
Having done this walk many times I would now recommend doing it counterclockwise. You get a nice flat stretch to warm up, a fairly steady climb to the top and then can enjoy the ocean views on the way down if the fog is cooperating.
Updates
San Francisco from Hill 88
Related Posts
- Golden Gate Park - Stow Lake, Strawberry Hill and Museum Concourse
- Lands End
- Coastal and Julian loop plus Black Sands Beach
- Milagra Ridge
- Fort Funston
(Hike Map)
(Published to the Fediverse as: Hill 88 #hike #hill88 #marinheadlands #sanfrancisco Marin Headlands hike up Hill 88, a loop of just under 5 miles and a little over 1,000 feet of elevation gain. )
Bernal Hill
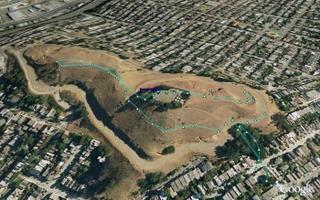
Closest walk from home is a quick trip round Bernal Hill for views of downtown San Francisco and three bridges.
(0.83 miles, total elevation gain 292 feet, 23 minutes (average 2.12 mph), view in Google Earth)
Hike starts at: 37.74355, -122.417583.
Related Posts
- Golden Gate Park - Stow Lake, Strawberry Hill and Museum Concourse
- Buena Vista Park
- Hill 88
- Golden Gate Park
- Oakland Hills
(Hike Map)
(Published to the Fediverse as: Bernal Hill #hike #bernal #sanfrancisco Hike around Bernal Hill in San Francisco, California (a little under a mile). )